Skb_tracer_arch
[TOC]
1. 前言
本文分析基于 5.4.0 内核,Ubuntu 20.04。
如果我们想了解 ip_rcv
被调用的堆栈路径,可以使用 BCC 中的 trace.py 工具获取;如果想了解 ip_rcv
函数内部调用的堆栈情况可以使用 funcgraph 工具查看。
BCC 安装参考 INSTALL.md。
相关命令使用样例。
|
|
2. Linux L2 层网络接收
2.1 软中断相关数据结构
删除了 RPS 和无关的成员变量。
struct softnet_data
为软中断处理结构,该结构为 per-CPU 变量,每个 CPU 具有一个单独的软中断数据结构。
|
|
2.2 Linux L2 层网络接收流程图
L2 与 L3 层的函数入口通过 packet_type
结构定义,对于 IPv4 协议对应的处理函数为 ip_rcv
。
|
|
3. Linux L3 层网络接收流程图
3.1 L3 层函数调用流程图
3.2 netfilter 相关结构
netfilter 协议和 hook 点对应的 hook 函数通过一个二维数据进行管理,在每个 Network Namespace 中都存在一个类似的二维数组。二维数组的纵轴为协议,横轴为 hook 点,两者交叉对应的数据为对应 hook 函数列表,按照优先级进行排列, hook 函数为各个 table 中设置的函数,初始化到对应的 hook 函数列表中。当初始化新的 network namespace 的时调用 iptable_nat_init
函数将对应的 table 初始化 (例如 iptable_nat_table_init),在 table 初始化函数中循环将 nf_xxx_ipv4_ops
结构中定义的 hook 函数进行注册。
hook 的函数定义为:
|
|
参考这里, 中文。表格代表了 iptables 的管理方式,按照 table 和 chain 的方式组织,纵轴代表的是 table 名,横轴是 chain 的名字,与 hook 点一一对应。纵轴的方向代表了在某个 chain 上调用的顺序,优先级自上而下。
Tables↓ /Chains→ | PREROUTING | INPUT | FORWARD | OUTPUT | POSTROUTING |
---|---|---|---|---|---|
(routing decision) | ✓ | ||||
raw | ✓ | ✓ | |||
(connection tracking enabled) | ✓ | ✓ | |||
mangle | ✓ | ✓ | ✓ | ✓ | ✓ |
nat (DNAT) | ✓ | ✓ | |||
(routing decision) | ✓ | ✓ | |||
filter | ✓ | ✓ | ✓ | ||
security | ✓ | ✓ | ✓ | ||
nat (SNAT) | ✓ | ✓ |
3.3 netfilter hook 函数调用
|
|
NF_HOOK 宏在启用 netfilter 的条件编译下,会首先调用 nf_hook
函数,在该函数中会根据参入的协议和 hook 点,获取到对应的 hook 函数列表头(例如 IPv4 协议中的 net->nf.hooks_ipv4[hook]
),然后在 nf_hook_slow
中循环调用列表中的 hook
函数(hook
函数按照优先级组织),并基于 hook
函数返回的结果决定继续调用列表中后续的 hook
函数,还是直接返回。
netfilter 中 hook
函数的格式基本如下,直接调用 ipt_do_table
函数,最后的参数传入对应的 table
字段。
|
|
所以,如果我们想要获取到 netfilter 的结果,则需要跟踪 ipt_do_table
函数的入参和返回结果即可。
|
|
参考
- [译] Linux 网络栈监控和调优:发送数据(2017) 👍
- [译] Linux 网络栈监控和调优:接收数据(2016) 👍
- [译] 深入理解 iptables 和 netfilter 架构 英文 👍
- Linux kernel 链路层帧接收 链路层输出 -qdisc
- linux协议栈调用流程
- Linux协议栈–Netfilter源码分析
- 图解Linux网络包接收过程 张彦飞 图解 Linux 网络包发送过程 👍
- 【梁金荣】当eBPF遇上Linux内核网络
- 浅析Linux内核网络子系统.pdf 提取码: ywpv 👍
CO-RE Clang/LLVM 10+ Ubuntu 20.10 (LLVM 11)
CO-RE 环境依赖 (内核 + Clang/LLVM 10+ )
参考
- HOWTO: BCC to libbpf conversion 如何将 bcc 的代码转成 CO-RE
- 官方参考样例 libbpf-tools in BCC repo contain lots of real-world tools converted from BCC to BPF CO-RE. Consider converting some more to both contribute to the BPF community and gain some more experience with it.
代码编写
-
Makefile 文件
-
libbpf-bootstrap
监控网络连接 CO-RE 程序编写
- BPF CO-RE 示例代码解析
- Analysis of BPF CO-RE sample code
- libbpf
- Ubuntu 20.10
- libbpf-bootstrap
- the companion blog post
- BPF Portability and CO-RE
- HOWTO: BCC to libbpf conversion
- libbpf-tools in BCC repo contain lots of real-world tools converted from BCC to BPF CO-RE. Consider converting some more to both contribute to the BPF community and gain some more experience with it.
- 原文作者:DavidDi
- 原文链接:https://www.ebpf.top/post/skb_tracer_arch/
- 版权声明:本作品采用知识共享署名-非商业性使用-禁止演绎 4.0 国际许可协议进行许可,非商业转载请注明出处(作者,原文链接),商业转载请联系作者获得授权。
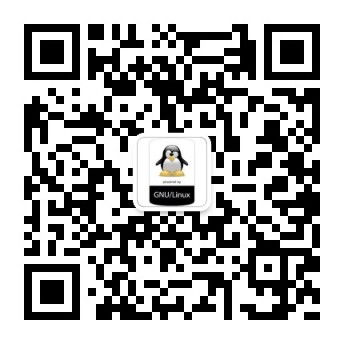
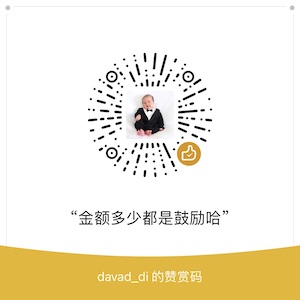